Pay with Google Pay™
Important
Google Pay is available to Android applications using the Svea App Wallet SDK from version 2.2.0.
Reach out to your Svea contact to verify active configuration on server.
Your partner application is responsible for integrating towards the Google Pay API. Use it to get a PaymentData
object representing the payment method selected by the user and convert it to GooglePayPaymentData
.
You will also need, as for all payments made with the Svea App Wallet SDK, a PaymentToken from your server.
Once you have both the PaymentToken andGooglePayPaymentData
, call payWithGooglePay()
.
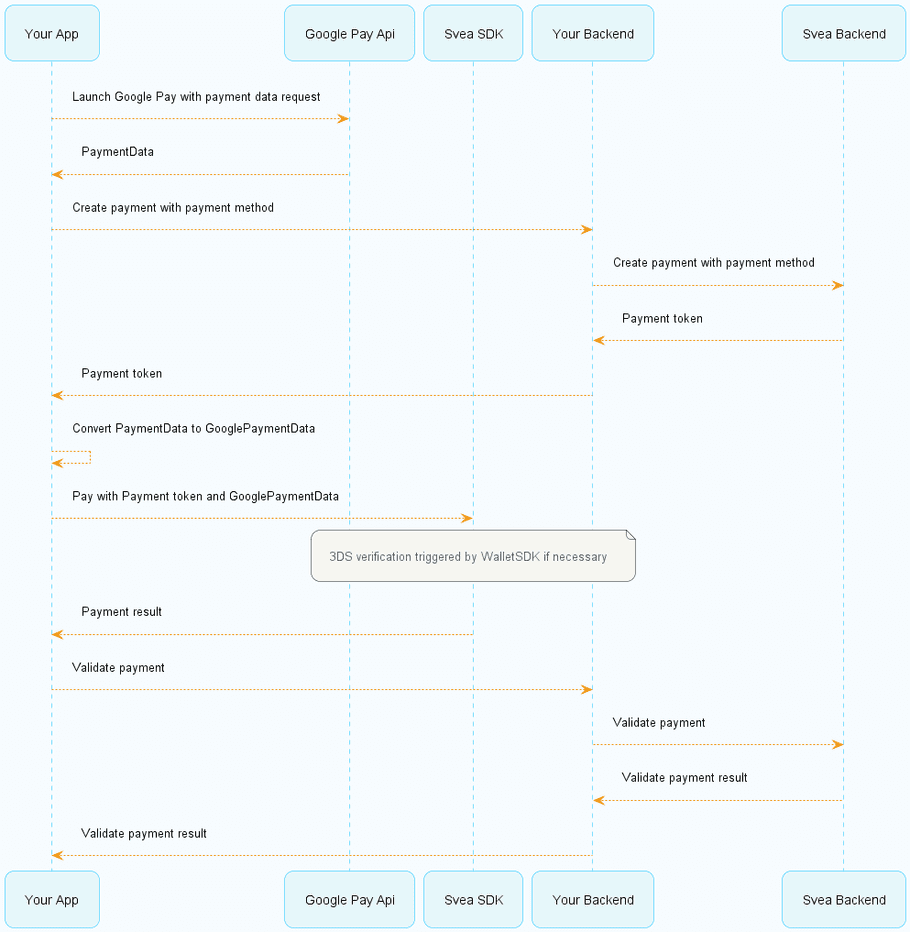
Integrate with Google Pay API
Below you will see a few necessary configurations as well as some pointers, but for detailed instructions please visit the official documentation.
Make sure to utilize the setup instructions, integration checklist and follow the brand guidelines.
Google Pay Configurations
To interact with the Google Pay API some base values need to be configured. Use the values specified below.
warning
Any code samples provided are on an "as is" basis, without warranty of any kind, either expressed, implied, or statutory, including, without limitation, warranties.
Tokenization
Key | Value |
---|---|
gateway | svea |
gatewayMerchantId | Reach out to your contact at Svea to receive a merchant id. |
private fun getGatewayTokenizationSpecification(): JSONObject {
return JSONObject().apply {
put("type", "PAYMENT_GATEWAY")
put(
"parameters", JSONObject(
mapOf(
"gateway" to "svea",
"gatewayMerchantId" to "your_merchant_id"
)
)
)
}
}
Allowed card networks and authentication methods
Key | Value |
---|---|
allowedCardNetworks | VISA, MASTERCARD |
allowedCardAuthMethods | PAN_ONLY, CRYPTOGRAM_3DS |
private val allowedCardNetworks = listOf("MASTERCARD", "VISA")
private val allowedCardAuthMethods = listOf("PAN_ONLY", "CRYPTOGRAM_3DS")
Verify Google Pay availability
When configured to support Google Pay, Google Pay will be returned as an option when querying getPaymentMethods()
.
Google only wants applications to show Google Pay as an option if it's a valid option and available on the device. Google has information about how to verify availability here. This means your partner app will have to verify that Google Pay is returned as a payment method option and Google Pay availability.
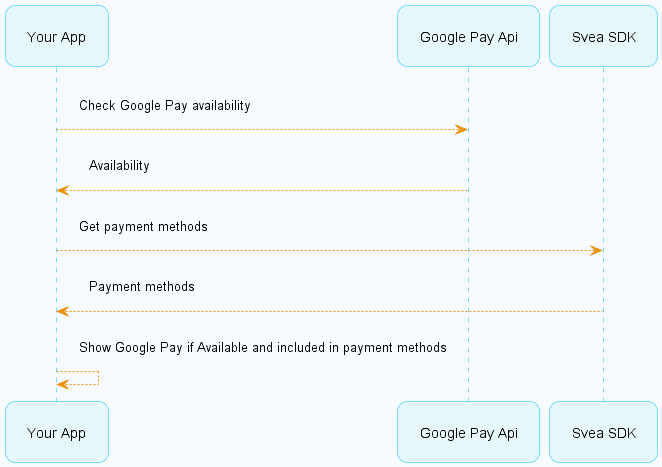
info
Google Pay will not be returned as a payment method in the Svea App Wallet SDK versions before 2.2.0.
Below are examples of how to handle the state.
In Kotlin we are using coroutines and combined StateFlows
, while the Java example is of a simpler kind.
private val googlePayAvailabilityFlow = MutableStateFlow(false)
private val unfilteredPaymentMethods = MutableStateFlow<List<PaymentMethod>>(emptyList())
private val availablePaymentMethods =
googlePayAvailabilityFlow.combine(unfilteredPaymentMethods) { googleAllowed, paymentMethods ->
if (!googleAllowed) paymentMethods.filter { it.type != PaymentType.GOOGLE_PAY }
else paymentMethods
}
...
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
checkGooglePayAllowed()
updatePaymentMethods()
lifecycleScope.launch {
availablePaymentMethods.collect { paymentMethods ->
//Update payment methods list
}
}
...
fun checkGooglePayAllowed() {
val isReadyToPayJson = buildIsReadyToPayRequest() ?: return
val request = IsReadyToPayRequest.fromJson(isReadyToPayJson.toString())
val task = googlePaymentsClient.isReadyToPay(request)
task.addOnCompleteListener { completedTask ->
try {
completedTask.getResult(ApiException::class.java)?.let {
lifecycleScope.launch {
googlePayAvailabilityFlow.emit(allowed)
}
}
} catch (exception: ApiException) {
// Process error
Log.w("Tag", "isReadyToPay failed.", exception)
lifecycleScope.launch {
googlePayAvailabilityFlow.emit(false)
}
}
}
}
...
private fun updatePaymentMethods() {
SveaWallet.getPaymentMethods(object : GetPaymentMethodsResult {
override fun onSuccess(paymentMethods: List<PaymentMethod>) {
lifecycleScope.launch {
unfilteredPaymentMethods.emit(paymentMethods)
}
}
override fun onFailure(failure: WalletError) {
Log.e("Tag", "Failed to get payment methods. \n $failure")
}
})
}
Converting PaymentData to GooglePayPaymentData
Once you have received a PaymentData
object
from Google Pay, convert it to a GooglePayPaymentData
object.
Here is a suggestion of how the conversion can be done.
val paymentData = PaymentData.getFromIntent(intent)
val paymentDataAsJson = paymentData.toJson()
val googlePayPaymentData =
Gson().fromJson(paymentDataAsJson, GooglePayPaymentData::class.java)
info
In stage
environment the supplied GooglePayPaymentData
will be changed to mocked object before being sent to the server.
Don't forget to
- Add Google Pay as dependency to your project
- Add metadata to your manifest
- Follow Google brand guidelines when showing a button
- Register in the Google Pay Business Console for production access
Perform payment
Once you have both a PaymentToken and the GooglePayPaymentData
object,
the user is able to make a payment with Google Pay.
info
If a payment needs to be verified with 3DSecure, then a web view will be shown to the user.
The Svea App Wallet SDK will automatically dismiss this view once the transaction is processed.
Parameter | Description |
---|---|
googlePayPaymentData | A GooglePayPaymentData object. |
paymentToken | A valid payment token from your backend. |
context | An application context |
onResult | Result callback handler. |
SveaWallet.payWithGooglePay(
googlePaymentData = googlePaymentData,
paymentToken = paymentToken,
context = applicationContext,
onResult = object : GooglePaymentCallback {
override fun onSuccess() {
print("Payment completed!")
}
override fun onFailure(failure: WalletError) {
print("Could not complete payment, got error: $failure")
}
})
import com.svea.wallet.domain.core.WalletError
SveaWallet.payWithGooglePay(
googlePaymentData = googlePaymentData,
paymentToken = paymentToken,
context = applicationContext,
onResult = object : GooglePaymentResult {
override fun onSuccess() {
print("Payment completed!")
}
override fun onFailure(failure: WalletError) {
print("Could not complete payment, got error: $failure")
}
})
onSuccess
Returned when the payment was successful.
Important
Please note that information about the purchase, such as the amount paid, is fetched from your backend.
onFailure
Returned when an error occurs. If this happens, the payment has failed.
Possible errors
object NoUserTokenProvided // A user token has not been provided.
class FailedToConfirmPayment // The payment could not be confirmed.
class PaymentFailed // The payment was not successful.
NO_PAYMENT_TOKEN_PROVIDED // A payment token has not been provided.
FAILED_TO_CONFIRM_PAYMENT // The payment could not be confirmed.
PAYMENT_FAILED // The payment was not successful.
UNKNOWN // An unknown error occurred.