Pay with Swish
Important
Introduced in Android version 2.5.0 and iOS version 3.3.0.
getSwishUrl
function using a payment token provided by your server.After obtaining this URL, the app should use it to create an intent and launch the Swish App.
The URLs are constructed in a way so that you should not need to process it before launching Swish from your app.
getPaymentResponse
in order to obtain the payment status.
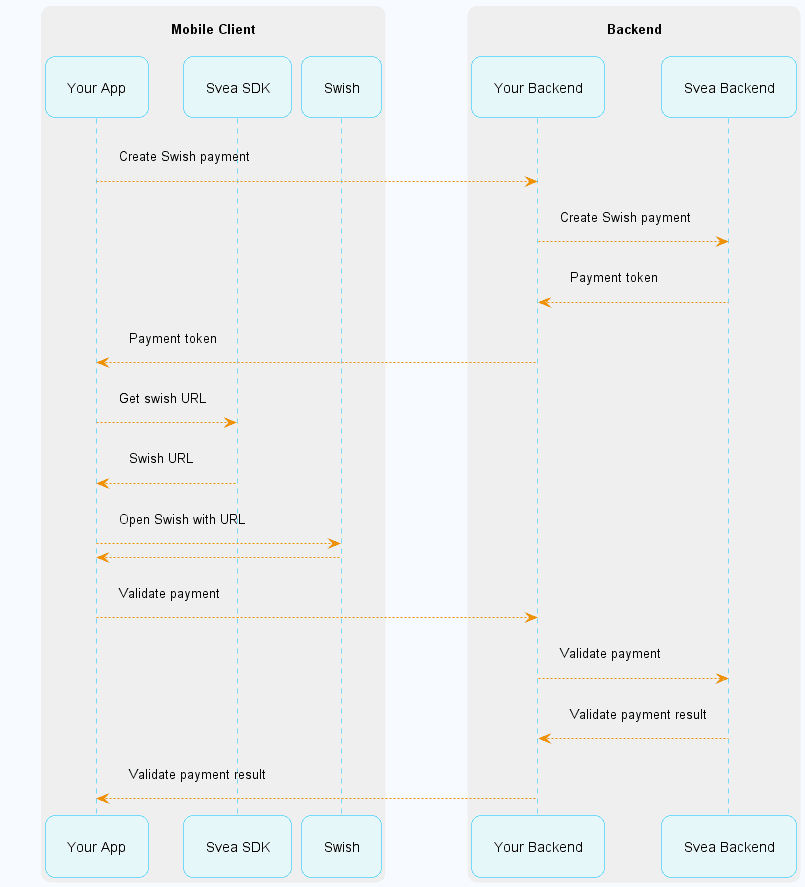
Please note
getSwishUrl
, the Android SDK returns an URI object for easier usage.Get Swish URL
Provides a valid Swish URL for this payment.
Parameter | Description |
---|---|
paymentToken | A valid payment token from your backend. |
callbackUrl | A valid URL for returning to the application. |
receiveOn | The dispatch queue used when invoking the result callback handler. Defaults to main. |
onResult | Result callback handler. |
SveaAppWallet.shared.getSwishUrl(paymentToken: paymentToken, callbackUrl: URL) { [weak self] result in
switch result {
case .success(let swishUrl):
// Using swish url open Swish if installed.
UIApplication.shared.open(swishUrl. options: [:], completionHandler: nil])
case .failure(let error):
print("Could not get swish url, got error: \(error)")
}
}
onSuccess
Returned when a URL to used to launch swish is successfully generated
onFailure
Returned when an error occurs.
Possible errors
case noPaymentTokenProvided // No payment token was found
case failedToGetSwishUrl // The request could not be completed.
Get payment response
Returns the status of a Swish payment
Parameter | Description |
---|---|
receiveOn | The dispatch queue used when invoking the result callback handler. Defaults to main. |
onResult | Result callback handler. |
Please note
SveaWalletClient
is deprecated in version 3.1.0. Use new class SveaAppWallet
instead.SveaAppWallet.shared.getSwishPaymentResponse { [weak self] result in
switch result {
case .success:
print("Swish payment completed!")
case .failure(let error):
print("Swish payment could not be completed, got error \(error)")
}
}
SveaWalletClient.shared.getSwishPaymentResponse { [weak self] result in
switch result {
case .onSuccess:
print("Swish payment completed!")
case .onFailure(let error):
print("Swish payment could not be completed, got error \(error)")
}
}
onSuccess
Returned when a Swish payment is successfully completed.
onFailure
Returned when an error occurs.
Possible errors
case noPaymentTokenProvided // No payment token was found
case failedToConfirmPaymentStatus // Payment failed
Test Swish payments
We provide several options to test Swish payments in the stage environment. Unless otherwise specified, Swish mock will be used for new projects.
Swish mock
Swish mock is a web app that that simulates the Swish client. When configured for Swish mock, the example code above will open Swish mock in the default browser, and you will get options to test different scenarios.
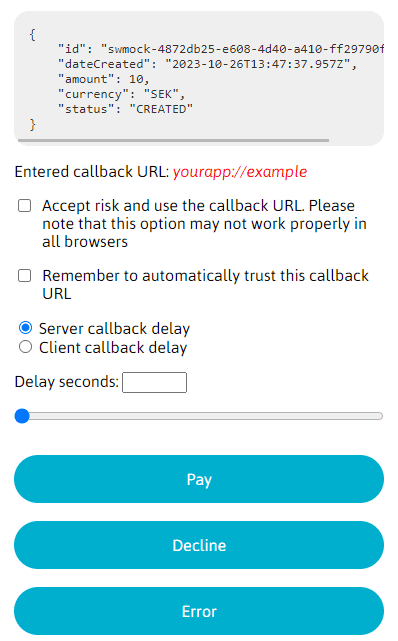
Complete payment
To complete a payment in Swish mock, press Pay, Decline or Error. This will result in the payment being completed with a corresponding status.
Note that the browser tab will stay open until you close it.
Test with callbackUrl
If you entered acallbackUrl
to your application you will get the option to use it when completing the payment.Note that some browsers will not work properly with this option
Test with callback delay
You can simulate delayed callbacks from either the Swish server or the Swish Client. This can be useful if you want to test your implementation for race conditions.
To use the client callback delay you must also use thecallbackUrl
. A server callback delay does not require the callbackUrl
, but you can use it for more precise timing.Fail to create a payment
By entering amount5.01
the payment will fail straight away, simulating an error from the Swish server.Merchant Swish Simulator
The official Merchant Swish Simulator can be used for testing without a Swish client.
Swish Sandbox
The official Swish Sandbox can be used for a more realistic test. Follow the link for instructions on how to set it up.